The Art of Clean Code: A Beginner’s Guide
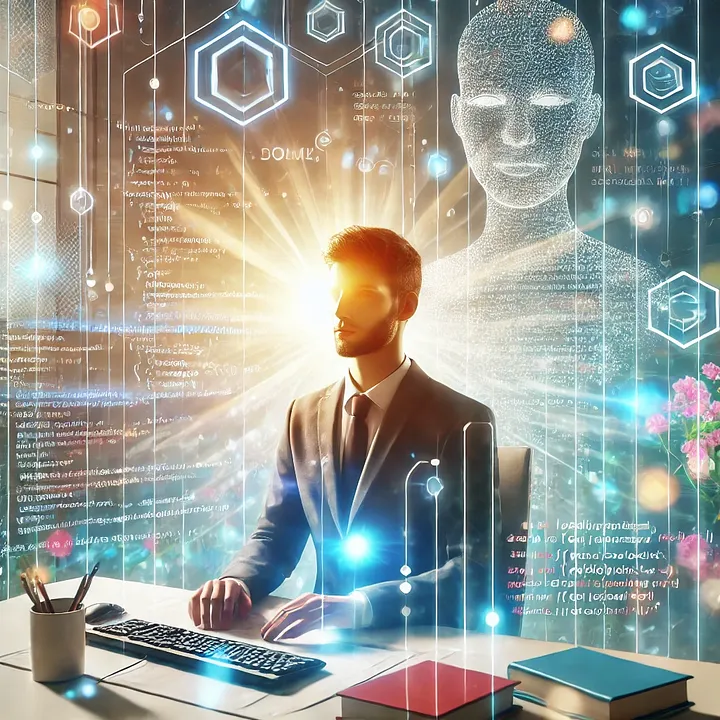
Writing clean code is a vital skill for any software developer. Clean code is easier to understand, maintain, and enhance, making it a cornerstone of professional software development. Whether you’re a seasoned coder or just starting, this guide will walk you through the principles and practices of writing clean code.
What is the Clean Code?
Clean code is code that is:
- Readable: Anyone can understand it without extensive explanations.
- Maintainable: Easy to modify and extend.
- Efficient: Performs well without unnecessary complexity.
- Testable: Facilitates unit and integration testing.
Why Clean Code Matters
During my programming journey, I’ve encountered many freshers who often struggle to write clean code. One common issue I noticed is that they focus on making the code “work” rather than making it “work well.” This leads to cluttered, hard-to-maintain code that slows down the entire development process.
Imagine trying to fix a bug in a piece of code you wrote six months ago. Even simple tasks can become nightmares if the code is cluttered and disorganized.
Clean code:
- Saves time during debugging.
- Reduces technical debt.
- Enhances collaboration within teams.
Common Mistakes Beginners Make
1. Overusing Comments
Many beginners write comments for every single line of code, explaining what is already obvious. Instead, focus on writing self-explanatory code and use comments sparingly to explain “why,” not “what.”
Mistake Example:
// Add 1 to i
i++;
Better Approach:
// Adjust index to start from 1 for user-facing display
index++;
2. Writing Monolithic Functions
Inexperienced developers often cram multiple responsibilities into a single function, making it hard to debug and test.
Mistake Example:
void ProcessData() {
// Validate input
// Process data
// Save data to database
// Log the operation
}
Better Approach:
void ValidateInput() { ... }
void ProcessData() { ... }
void SaveToDatabase() { ... }
void LogOperation() { ... }
3. Using Magic Numbers and Strings
Hardcoding values make the code less readable and harder to maintain.
Mistake Example:
if (age > 18) {
// Allow access
}
Better Approach:
const int LegalAge = 18;
if (age > LegalAge) {
// Allow access
}
Principles of Clean Code
1. Meaningful Names
Use descriptive and specific names for variables, functions, and classes.
Bad Example:
int d; // What does 'd' mean?
Good Example:
int daysSinceLastUpdate;
2. Functions Should Do One Thing
A function should have a single responsibility.
Bad Example:
void ProcessAndLogUserData() {
// Process data
// Log data
}
Good Example:
void ProcessUserData() {
// Process data
}
void LogUserData() {
// Log data
}
Learn more about the Single Responsibility Principle here.
3. Consistent Formatting
Ensure your code follows a uniform style for readability. Use tools like Prettier or EditorConfig to enforce consistent formatting.
Practices for Writing Clean Code
1. Refactor Regularly
Refactoring improves code structure without changing its functionality. I’ve often found that refactoring poorly written code from freshers can significantly reduce bugs and improve performance. Learn more about refactoring from Martin Fowler’s guide.
2. Write Tests
Testing ensures your code behaves as expected and makes future changes safer.
- Use unit tests for individual components.
- Employ integration tests for combined modules.
Explore JUnit for Java or NUnit for .NET.
3. Embrace DRY (Don’t Repeat Yourself)
Avoid duplicating code by creating reusable functions or components.
Bad Example:
void PrintUser() {
Console.WriteLine("User: John Doe");
}
void PrintAdmin() {
Console.WriteLine("Admin: John Doe");
}
Good Example:
void PrintRole(string role) {
Console.WriteLine($"{role}: John Doe");
}
4. Leverage Code Reviews
Collaborate with teammates to identify issues and improve code quality. Check out this guide to effective code reviews.
Tools to Help You Write Clean Code
- Linters: Tools like ESLint and StyleCop enforce coding standards.
- IDEs: Modern IDEs like Visual Studio and JetBrains Rider offer refactoring tools and suggestions.
- Code Analysis Tools: Use SonarQube to identify code smells and vulnerabilities.
Conclusion
Clean code is a journey, not a destination. Start small by applying these principles in your daily coding routine. With time, writing clean and efficient code will become second nature.
Remember, writing clean code is not just about impressing your peers — it’s about creating software that stands the test of time. And as I’ve learned, helping freshers understand these concepts early on can save countless hours of debugging and frustration.
For more in-depth reading, explore these resources:
Recent Posts
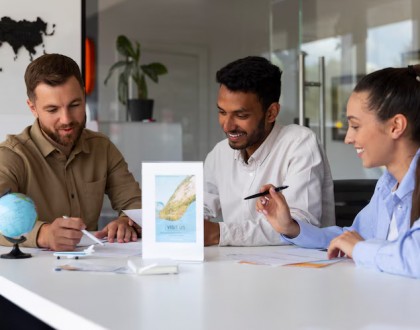
Shopify Migration Guide for Indian SMEs
June 26, 2025
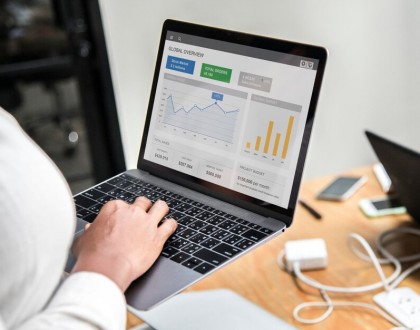
Shopify Analytics & Funnels for Conversions
June 20, 2025