Implementing Google reCAPTCHA
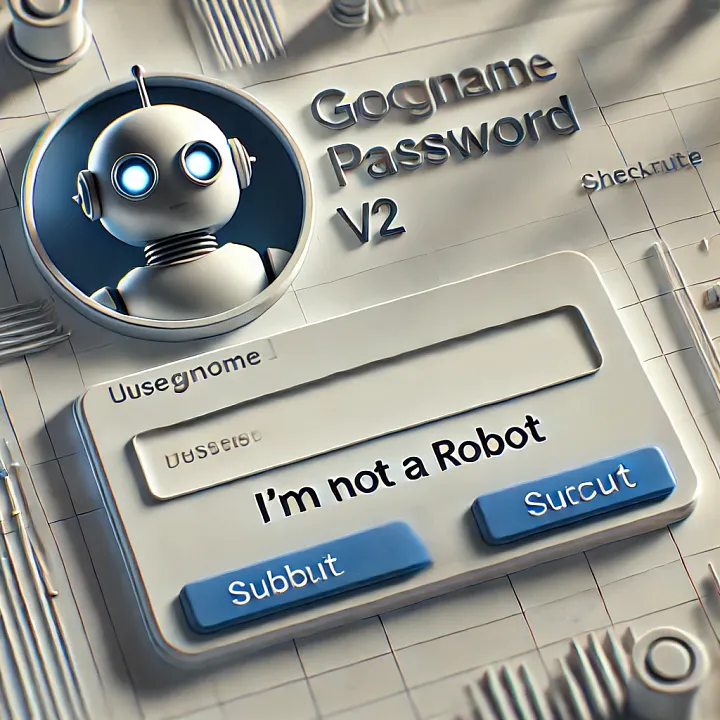
Google reCAPTCHA is a free service designed to protect websites from spam and abuse. It uses advanced risk analysis techniques to distinguish between human users and bots, ensuring that only genuine visitors interact with your website.
Why Use Google reCAPTCHA?
- Prevent Spam Submissions: Protects your web forms (e.g., contact forms, login, and registration) from automated bot attacks that flood them with spammy or malicious data.
- Enhanced Security: Stops bots from exploiting vulnerabilities in your website, reducing the risk of automated attacks like brute force or credential stuffing.
- User-Friendly: With reCAPTCHA v2’s “I am not a robot” checkbox, users find it simple to verify themselves without completing unnecessary challenges unless deemed suspicious.
- Cost-Free Protection: It’s a completely free service offered by Google, making it accessible to all websites.
Real-Life Use Cases
Contact Forms: Stop spam emails from being sent via automated bots.
Login Forms: Protect against brute-force attacks by ensuring only humans can access.
Online Registration Forms: Prevent fake account creation or abuse by malicious bots.
By implementing reCAPTCHA v2, you secure your website from potential threats while maintaining a user-friendly experience for real visitors.
Steps to Integrate Google reCAPTCHA v2
- Get reCAPTCHA API Keys
- Go to the Google reCAPTCHA Admin Console.
- Register your site and get the Site Key and Secret Key.
2. Add reCAPTCHA to Your page
<!–Include script block –>
<script src=“https://www.google.com/recaptcha/api.js” async defer></script>
<!–Now somewhere in body –>
<!– Google reCAPTCHA widget –>
<div class=“g-recaptcha” data-sitekey=“YOUR_SITE_KEY”></div>
3. Server-Side Verification (C# )
string recaptchaResponse = Request.Form["g-recaptcha-response"];
if (string.IsNullOrEmpty(recaptchaResponse))
{
lblMessage.Text = "Please complete the CAPTCHA.";
return;
}
// Verify reCAPTCHA
bool isValid = ValidateRecaptcha(recaptchaResponse);
if (isValid)
{
lblMessage.ForeColor = System.Drawing.Color.Green;
lblMessage.Text = "Form submitted successfully!";
// Process the form data (e.g., save to DB)
}
else
{
lblMessage.Text = "CAPTCHA validation failed. Please try again.";
}
}
private bool ValidateRecaptcha(string recaptchaResponse)
{
const string secretKey = "YOUR_SECRET_KEY";
string apiUrl = $"https://www.google.com/recaptcha/api/siteverify?secret={secretKey}&response={recaptchaResponse}";
try
{
// Send request to Google reCAPTCHA server
using (WebClient client = new WebClient())
{
string jsonResult = client.DownloadString(apiUrl);
// Parse JSON response
JavaScriptSerializer js = new JavaScriptSerializer();
RecaptchaResponse response = js.Deserialize(jsonResult);
// Return the success status
return response.Success;
}
}
catch (Exception ex)
{
// Log exception
lblMessage.Text = $"Error: {ex.Message}";
return false;
}
}
public class RecaptchaResponse
{
public bool Success { get; set; }
public string Challenge_ts { get; set; } // Timestamp of the challenge load
public string Hostname { get; set; } // Hostname of the site where the challenge was solved
public string[] ErrorCodes { get; set; } // Optional
}
Notes:
- Replace
YOUR_SITE_KEY
andYOUR_SECRET_KEY
with the keys you got from Google reCAPTCHA admin. - Ensure your website is hosted on a domain registered in the reCAPTCHA admin console.
- If you are testing in localhost, add the domain “localhost” in settings page of Google reCAPTCHA
- If your site runs on HTTPS, ensure the reCAPTCHA script (
https://www.google.com/recaptcha/api.js
) is loaded securely.
In conclusion, integrating Google reCAPTCHA v2 into your ASP.NET application is an essential step to enhance security and safeguard your website from spam and bot attacks. Its ease of integration, combined with robust protection and a user-friendly interface, ensures a seamless experience for genuine users while keeping malicious entities at bay. By implementing this simple yet powerful solution, you not only secure your web forms but also build trust and credibility with your users, reinforcing your commitment to providing a safe and reliable online platform.
Its so simple! right. Do implement and let us know in comment if any support needed.
Thanks for reading !
Recommended Posts
Turn Your Database into API with Data API Builder
March 25, 2025