Building Secure APIs: Best Practices Every Developer Should Follow
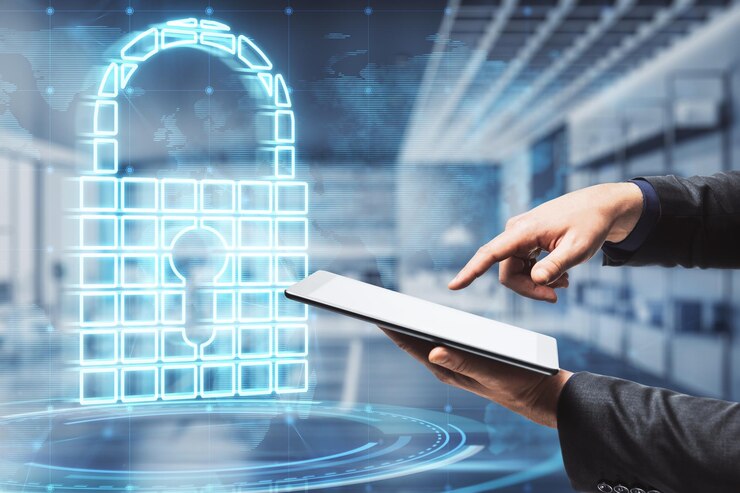
In my journey as a software developer, I’ve seen many APIs that are functional but lack security measures. API security is not something you can afford to overlook — especially when handling sensitive user data. Here, I’ll share some of the most effective security best practices for building APIs, along with real-world examples and external references to help you dive deeper.
1. Use Authentication and Authorization
APIs should always verify who is making the request and what they are allowed to do. This is where authentication (who you are) and authorization (what you can do) come into play.
How to Implement It:
- Use OAuth 2.0 for user authentication. (Read more about OAuth)
- Use JWT (JSON Web Tokens) for stateless authentication.
- Implement Role-Based Access Control (RBAC) to limit access based on roles.
Example: JWT Authentication in ASP.NET
var tokenHandler = new JwtSecurityTokenHandler();
var key = Encoding.ASCII.GetBytes("YourSecretKey");
var tokenDescriptor = new SecurityTokenDescriptor
{
Subject = new ClaimsIdentity(new[] { new Claim("id", user.Id.ToString()) }),
Expires = DateTime.UtcNow.AddHours(1),
SigningCredentials = new SigningCredentials(new SymmetricSecurityKey(key), SecurityAlgorithms.HmacSha256Signature)
};
var token = tokenHandler.CreateToken(tokenDescriptor);
var tokenString = tokenHandler.WriteToken(token);
2. Use HTTPS
Never expose your API over plain HTTP. Always enforce HTTPS to protect data in transit from being intercepted.
How to Implement It:
- Use SSL/TLS encryption.
- Redirect all HTTP requests to HTTPS.
Example: Enforce HTTPS in ASP.NET Core
public void ConfigureServices(IServiceCollection services)
{
services.AddHttpsRedirection(options =>
{
options.HttpsPort = 443;
});
}
More on enforcing HTTPS: OWASP Guide to Transport Layer Security
3. Implement Rate Limiting
To prevent API abuse (e.g., DDoS attacks), limit the number of requests a client can make.
How to Implement It:
- Use API Gateway (like NGINX, Kong, or AWS API Gateway).
- Implement rate limiting using middleware.
Example: Rate Limiting in ASP.NET Core
services.AddRateLimiter(options =>
{
options.AddPolicy("fixed",
new FixedWindowRateLimiterPolicy(100, TimeSpan.FromMinutes(1)));
});
Read more about rate limiting best practices.
4. Validate and Sanitize User Input
User input is one of the biggest security risks. Always validate and sanitize incoming data to prevent SQL injection, XSS, and command injection.
How to Implement It:
- Use parameterized queries to prevent SQL injection.
- Validate input length, format, and expected values.
- Use libraries like OWASP Java Encoder or Microsoft AntiXSS.
Example: Prevent SQL Injection in C#
using (var command = new SqlCommand("SELECT * FROM Users WHERE Email = @Email", connection))
{
command.Parameters.AddWithValue("@Email", userInput);
var reader = command.ExecuteReader();
}
More on SQL Injection Prevention.
5. Log and Monitor API Activity
Logging helps in debugging, security audits, and identifying potential attacks.
How to Implement It:
- Use structured logging (e.g., Serilog, ELK Stack).
- Implement real-time monitoring with tools like Prometheus and Grafana.
Example: Logging API Requests in ASP.NET Core
app.Use(async (context, next) =>
{
var request = await new StreamReader(context.Request.Body).ReadToEndAsync();
Log.Information("Received request: {request}", request);
await next();
});
6. Use Security Headers
Security headers add an extra layer of protection against common attacks.
How to Implement It:
- Use Content Security Policy (CSP) to prevent XSS.
- Implement X-Frame-Options to prevent clickjacking.
- Use Strict-Transport-Security (HSTS).
Example: Add Security Headers in ASP.NET Core
app.Use(async (context, next) =>
{
context.Response.Headers.Add("X-Content-Type-Options", "nosniff");
context.Response.Headers.Add("X-Frame-Options", "DENY");
context.Response.Headers.Add("X-XSS-Protection", "1; mode=block");
await next();
});
More on security headers.
Conclusion
Securing an API is not a one-time effort — it’s a continuous process. By following these best practices — authentication, HTTPS, rate limiting, input validation, logging, and security headers — you can significantly reduce vulnerabilities.
If you’re building APIs, start implementing these today and explore more security principles on OWASP API Security.
Have you faced any security issues in API development? Let me know in the comments!
Recommended Posts
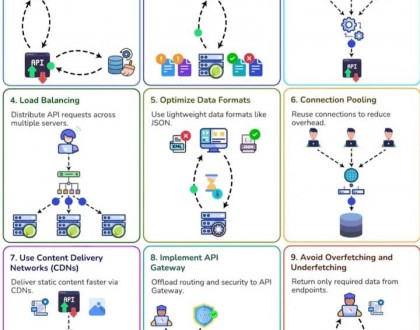
Key Strategies to Boost API Performance in ASP.net
March 10, 2025